让多个组件使用同一个挂载点,并动态切换,这就是动态组件。
常规实现方案
使用v-if来实现子组件之间的切换,使用一个索引控制,当索引匹配成功时组件显示(使用v-show也可以)
- v-if:v-if 控制元素显示或隐藏是把dom元素整个的渲染或者删除
- v-show:改变css样式display控制显示隐藏
回到正题,引入三个组件,然后使用v-if控制显示隐藏
<template lang="pug">
.steps
el-steps(:active="active", finish-status="success")
el-step(title="步骤 1")
el-step(title="步骤 2")
el-step(title="步骤 3")
.son(v-if="active === 1")
component1
.son(v-if="active === 2")
component2
.son(v-if="active === 3")
component3
el-button(style="margin-top: 12px;", @click="next") 下一步
el-button(style="margin-top: 12px;", @click="pre", v-if="active > 0") 上一步
</template>
<script>
import component1 from '../components/component1'
import component2 from '../components/component2'
import component3 from '../components/component3'
export default {
components: {
component1,
component2,
component3,
},
data() {
return {
active: 0,
};
},
methods: {
next() {
if (this.active++ > 2) this.active = 0;
},
pre() {
if (this.active-- < 1) this.active = 0;
},
},
};
</script>
效果
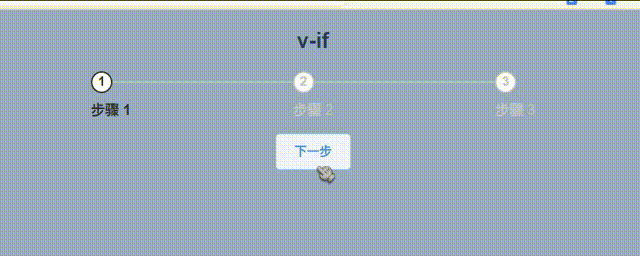
内置组件component
试过组件id来控制显示哪个组件,先将组件名放在数组中,通过索引获取数组中的组件名来绑定组件,使用计算属性监听,当索引发生变化时,计算属性的值随之改变
看代码
<template lang="pug">
.steps
h2 component
el-steps(:active="active", finish-status="success")
el-step(title="步骤 1")
el-step(title="步骤 2")
el-step(title="步骤 3")
component(:is="componentId")
el-button(style="margin-top: 12px;", @click="next") 下一步
el-button(style="margin-top: 12px;", @click="pre", v-if="active > 0") 上一步
</template>
<script>
import component1 from '../components/component1'
import component2 from '../components/component2'
import component3 from '../components/component3'
export default {
components: {
component1,
component2,
component3,
},
data() {
return {
active: 0,
componentArr: ['component1', 'component2', 'component3']
};
},
computed: {
componentId() {
return this.componentArr[this.active]
}
},
methods: {
next() {
if (this.active++ > 2) this.active = 0;
},
pre() {
if (this.active-- < 1) this.active = 0;
},
},
};
</script>
</style>
效果
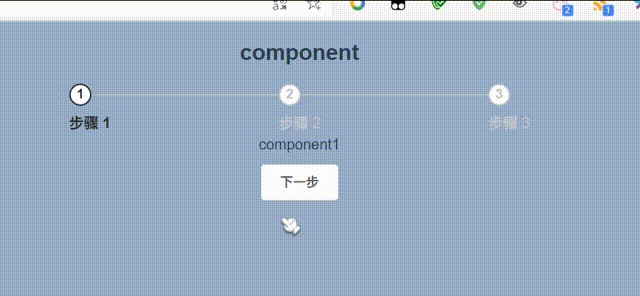
可以看出两种方式实现的效果基本无差异,如果动态组件涉及表单,可以使用keep-alive缓存表单内容